- Think Ahead With AI
- Posts
- ๐ Is Quantum AI the Future? How to Build a Model with Python! ๐
๐ Is Quantum AI the Future? How to Build a Model with Python! ๐
Dive into the Quantum Realm and Discover How to Supercharge AI with Quantum Computing! ๐
Story Highlights ๐ก๐ฌ
โจ Quantum computing's potential to revolutionize AI.
๐ Python code example for building a quantum AI model.
โก๏ธ Overcoming deep learning's energy challenges.

The 5 Ws of Quantum AI ๐ค
Who:๐ฉโ๐ป Tech enthusiasts, AI developers, quantum computing researchers.
What:๐ Exploring the intersection of quantum computing and AI.
When:๐ฐ๏ธ The era of quantum computing is now emerging.
Where:๐ Across the globe, in research labs, universities, and tech companies.
Why:โ๏ธ To enhance computational efficiency and tackle deep learning's energy demands.
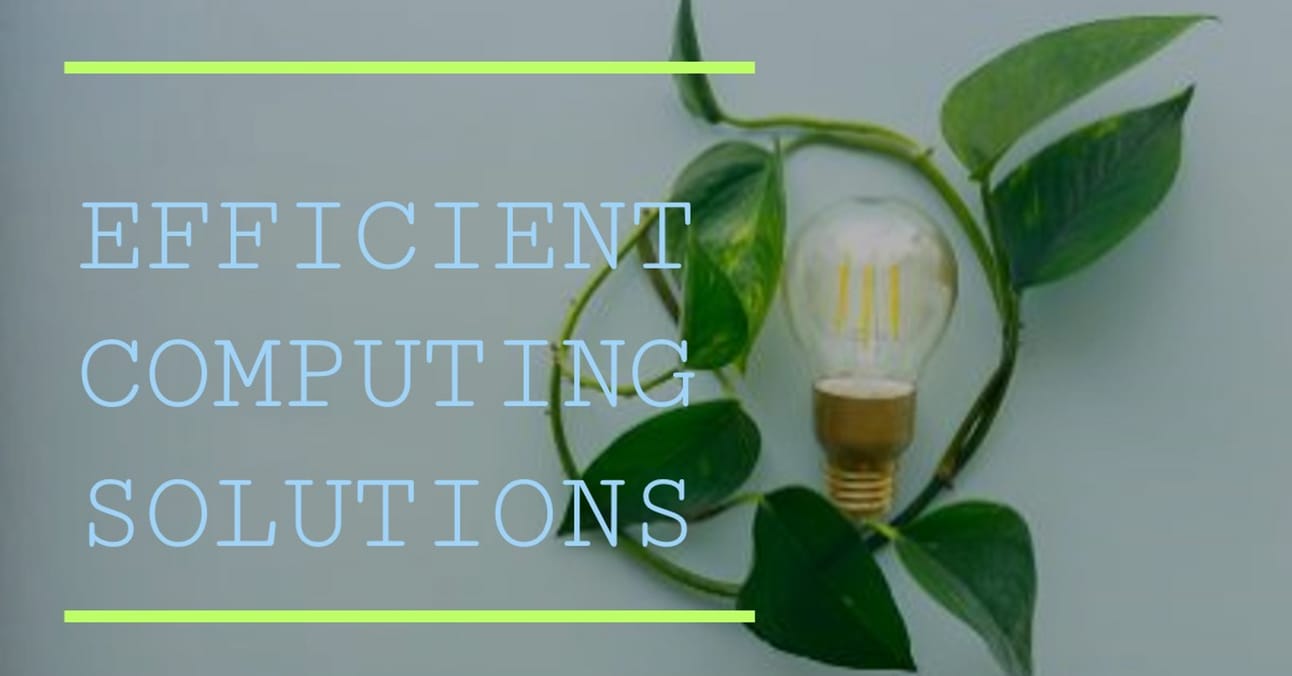
Artificial Intelligence and the Emergence of Deep Learning ๐ค
What is Deep Learning in Artificial Intelligence? ๐ง
Deep learning, a subset of AI, employs neural networks to decipher complex patterns, akin to the strategies a sports team uses to win a match.
The larger the neural network, the more capable it becomes of performing impressive featsโsuch as ChatGPT, which uses natural language processing to interact with users seamlessly.

At the heart of deep learning is the training of neural networks. This process involves using data to adjust the values of each neuron, enabling the network to make accurate predictions.
Neurons are organized into layers, with each layer extracting unique features from the data. This structure allows deep learning models to analyze and interpret intricate data effectively.
The Challenge of Computational Efficiency in Deep Learning โก
Despite its transformative impact on society, deep learning is plagued by a critical issue: computational efficiency.
Training deep learning models demands immense data and computational power, which can take anywhere from minutes to weeks, consuming substantial energy and resources.
Solutions to this problem include improving algorithmic efficiency and enhancing computational efficiency.

Quantum computing is one of the promising solutions that address the latter.
A Potential Solution: Quantum Computing ๐ก
Why Quantum Computing Could Be a Game-Changer ๐
Quantum computing offers a promising avenue for addressing the computational efficiency challenges in deep learning.
Unlike classical computers that operate with bits (0 or 1), quantum computers use qubits, which can represent 0 and 1 simultaneously due to a phenomenon known as superposition.
This capability allows quantum computers to process numerous possibilities concurrently, making them more efficient for specific tasks than classical computers.

Quantum-based algorithms can significantly reduce the energy consumption associated with AI model creation.
Why Are Quantum Computers Not So Widely Used? ๐ค
The primary challenge in quantum computing lies in the physical representation of qubits. While classical bits are managed using transistors, qubits are created using superconducting circuits, trapped ions, and topological qubitsโall of which are costly.

However, companies like IBM and Amazon are making strides by providing cloud-based access to quantum computing resources, enabling researchers and developers to experiment with quantum algorithms.
Code Example: A Quantum AI Model for Quantum Chemistry ๐ป
What is Quantum Chemistry? ๐ฌ
Quantum chemistry examines how electrons behave in atoms and molecules, using quantum physics to understand the interactions that form various chemical substances. Our goal is to find the "ground state energy" of the Hโ molecule, which represents the molecule's most stable form and properties.

Classical computers struggle with this problem due to the vast number of possibilities and intricate interactions involved.
Quantum computers, however, can simulate these interactions directly, making them well-suited for such tasks.
Approximating with the Variational Quantum Eigensolver (VQE) ๐
The Variational Quantum Eigensolver (VQE) is a hybrid algorithm that combines quantum and classical computing.
In our example, we'll use the VQE algorithm to find the ground state energy of the Hโ molecule. The code is designed to run on a quantum simulator but can be adapted for actual quantum hardware through a cloud-based service.
Letโs go through the code step by step:
PYTHON CODE
import pennylane as qml
import numpy as np
import matplotlib.pyplot as plt
# Define the molecule (H2 at bond length of 0.74 ร
)
symbols = ["H", "H"]
coordinates = np.array([0.0, 0.0, 0.0, 0.0, 0.0, 0.74])
# Generate the Hamiltonian for the molecule
hamiltonian, qubits = qml.qchem.molecular_hamiltonian(
symbols, coordinates)
# Define the quantum device
dev = qml.device("default.qubit", wires=qubits)
# Define the ansatz (variational quantum circuit)
def ansatz(params, wires):
qml.BasisState(np.array([0] * qubits), wires=wires)
for i in range(qubits):
qml.RY(params[i], wires=wires[i])
for i in range(qubits - 1):
qml.CNOT(wires=[wires[i], wires[i + 1]])
# Define the cost function
@qml.qnode(dev)
def cost_fn(params):
ansatz(params, wires=range(qubits))
return qml.expval(hamiltonian)
# Set a fixed seed for reproducibility
np.random.seed(42)
# Set the initial parameters
params = np.random.random(qubits, requires_grad=True)
# Choose an optimizer
optimizer = qml.GradientDescentOptimizer(stepsize=0.4)
# Number of optimization steps
max_iterations = 100
conv_tol = 1e-06
# Optimization loop
energies = []
for n in range(max_iterations):
params, prev_energy = optimizer.step_and_cost(cost_fn, params)
energy = cost_fn(params)
energies.append(energy)
if np.abs(energy - prev_energy) < conv_tol:
break
print(f"Step = {n}, Energy = {energy:.8f} Ha")
print(f"Final ground state energy = {energy:.8f} Ha")
# Visualize the results
iterations = range(len(energies))
plt.plot(iterations, energies)
plt.xlabel('Iteration')
plt.ylabel('Energy (Ha)')
plt.title('Convergence of VQE for H2 Molecule')
plt.show()
OUTPUT
This code is a quantum simulation using PennyLane to find the ground state energy of an H2 molecule with a bond length of 0.74 ร . It uses the Variational Quantum Eigensolver (VQE) algorithm to optimize the energy and visualize the convergence of the optimization process.

This code snippet demonstrates a variational quantum eigensolver (VQE) to determine the ground state energy of a hydrogen molecule (H2) using the PennyLane quantum computing library.
Letโs break it down:
1. Imports
import pennylane as qml
import numpy as np
import matplotlib.pyplot as plt
- pennylane: Quantum computing library used for variational algorithms and quantum machine learning.
- numpy: Library for numerical operations, such as handling arrays.
- matplotlib.pyplot:** Library for plotting graphs.
2. Define the Molecule
symbols = ["H", "H"]
coordinates = np.array([0.0, 0.0, 0.0, 0.0, 0.0, 0.74])
- symbols: List of atomic symbols representing the molecule (Hydrogen in this case).
- coordinates: 3D coordinates of the atoms. For H2 at a bond length of 0.74 ร
, it's specified in a flat array for two hydrogen atoms.
3. Generate the Hamiltonian
hamiltonian, qubits = qml.qchem.molecular_hamiltonian(symbols, coordinates)
- qml.qchem.molecular_hamiltonian: A PennyLane function that generates the Hamiltonian matrix for the given molecule, which represents the energy of the system in quantum mechanics. It also returns the number of qubits required to represent the molecule.
4. Define the Quantum Device
dev = qml.device("default.qubit", wires=qubits)
- qml.device: Specifies the quantum device to use. Here, `default.qubit` is a simulator for running quantum circuits, and `wires=qubits` indicates the number of qubits.
5. Define the Ansatz (Variational Circuit)
def ansatz(params, wires):
qml.BasisState(np.array([0] * qubits), wires=wires)
for i in range(qubits):
qml.RY(params[i], wires=wires[i])
for i in range(qubits - 1):
qml.CNOT(wires=[wires[i], wires[i + 1]])
- ansatz: The quantum circuit used in the VQE algorithm. It consists of:
- BasisState: Initializes the qubits to the |0โฉ state.
- RY gates: Rotate qubits around the Y-axis with parameters. This introduces variability into the quantum state.
- CNOT gates: Entangle adjacent qubits, adding complexity to the quantum state.
6. Define the Cost Function
@qml.qnode(dev)
def cost_fn(params):
ansatz(params, wires=range(qubits))
return qml.expval(hamiltonian)
- @qml.qnode: A decorator that creates a quantum node for the cost function, which runs on the quantum device.
- cost_fn: This function evaluates the energy expectation value of the Hamiltonian given the parameters of the ansatz circuit.
7. Initialize Parameters and Optimizer
np.random.seed(42)
params = np.random.random(qubits, requires_grad=True)
optimizer = qml.GradientDescentOptimizer(stepsize=0.4)
- np.random.seed(42): Sets the random seed for reproducibility.
- params: Initial parameters for the ansatz circuit, randomly generated.
- optimizer: Optimization algorithm used to minimize the cost function. `GradientDescentOptimizer` is chosen with a step size of 0.4.
8. Optimization Loop
max_iterations = 100
conv_tol = 1e-06
energies = []
for n in range(max_iterations):
params, prev_energy = optimizer.step_and_cost(cost_fn, params)
energy = cost_fn(params)
energies.append(energy)
if np.abs(energy - prev_energy) < conv_tol:
break
print(f"Step = {n}, Energy = {energy:.8f} Ha")
print(f"Final ground state energy = {energy:.8f} Ha")
- max_iterations: Maximum number of iterations for optimization.
- conv_tol: Convergence tolerance; the loop stops if energy change is less than this value.
- energies: List to track energy values over iterations.
- optimizer.step_and_cost: Updates parameters and computes the new cost.
- The loop continues until either the maximum number of iterations is reached or convergence is achieved.
9. Visualize the Results
iterations = range(len(energies))
plt.plot(iterations, energies)
plt.xlabel('Iteration')
plt.ylabel('Energy (Ha)')
plt.title('Convergence of VQE for H2 Molecule')
plt.show()
- plt.plot: Plots the energy values versus iterations.
- plt.xlabel, plt.ylabel, plt.title: Labels and titles the plot.
- plt.show(): Displays the plot, showing how the energy converges over iterations.
This entire process demonstrates how VQE can be used to approximate the ground state energy of a molecule by optimizing the parameters of a quantum circuit.
Key Points: ๐ฌ
๐ Initial Energy: Approximately 1.4 Ha at iteration 0.
๐ Rapid Decrease: Energy quickly drops within the first 20 iterations.
๐ Plateau: Energy stabilizes around 0.4 Ha after 20 iterations, indicating convergence to an optimal or near-optimal solution.
Conclusion: The Limits and Future of Quantum Computing ๐
Quantum computing holds the potential to revolutionize deep learning by addressing the issue of computational efficiency.
However, it remains in the experimental phase and requires further development before it becomes mainstream. Companies like IBM and Amazon are paving the way by providing cloud-based access to quantum resources, enabling researchers and developers to experiment with quantum algorithms.

As quantum technology continues to advance, its integration with AI will likely bring about transformative changes in the field of machine learning.
Why It Matters and What You Should Do ๐ค๐ก
๐ค Understand the potential of quantum computing to transform AI and machine learning.
๐ค Experiment with quantum computing through cloud-based platforms like IBM and Amazon.
๐ค Stay informed about the latest developments in quantum technology.
๐ค Explore Python libraries like PennyLane for hands-on practice with quantum circuits.
โThe only way to discover the limits of the possible is to go beyond them into the impossible."
Generative AI Tools ๐ง
๐ฅ Hemingway Editor Plus: Fix wordy sentences, grammar issues, and more with the help of AI.
๐ค FlexClip: Use AI to create clips smarter and faster โ now with a background noise removal feature.
๐ฉ๐ผโ๐ฆฐ Lately: The worldโs first Deep Social platform powered by Neuroscience-Driven AIโข. Elevate your content from meh to WOW - faster than you can grab a coffee โ
๐ Tern: Input your travel preferences, then receive a custom itinerary thatโs perfectly suited to your tastes.
โ๏ธ Airtable Cobuilder: Instantly create an app that connects and streamlines your most critical data.
News ๐ฐ
About Think Ahead With AI (TAWAI) ๐ค

Empower Your Journey With Generative AI.
"You're at the forefront of innovation. Dive into a world where AI isn't just a tool, but a transformative journey. Whether you're a budding entrepreneur, a seasoned professional, or a curious learner, we're here to guide you."
Think Ahead With AI is more than just a platform.
Founded with a vision to democratize Generative AI knowledge,
It's a movement.
Itโs a commitment.
Itโs a promise to bring AI within everyone's reach.
Together, we explore, innovate, and transform.
Our mission is to help marketers, coaches, professionals and business owners integrate Generative AI and use artificial intelligence to skyrocket their careers and businesses. ๐
TAWAI Newsletter By:

Sujata Ghosh
Gen. AI Explorer
โTAWAI is your trusted partner in navigating the AI Landscape!โ ๐ฎ๐ช